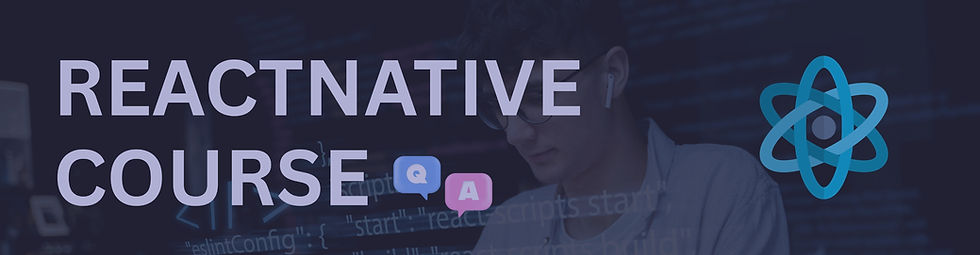
React Native Interview Questions and Answers
Top 100 React Native Interview Questions for Freshers
React Native is one of the most in-demand skills in top tech companies, including IDM TechPark. Mastering cross-platform mobile development, component-based UI design, state management, and performance optimization makes a React Native Developer a valuable asset in modern mobile app development.
To secure a React Native Developer role at IDM TechPark, candidates must be proficient in technologies like JavaScript, TypeScript, React, Redux, Expo, Firebase, and native modules, as well as be prepared to tackle both the React Native Online Assessment and Technical Interview Round.
To help you succeed, we have compiled a list of the Top 100 React Native Interview Questions along with their answers. Mastering these will give you a strong edge in cracking React Native interviews at IDM TechPark
1. What is React Native?
Answer:
React Native is an open-source framework developed by Facebook that allows developers to build mobile applications using JavaScript and React. It enables cross-platform development, meaning you can write one codebase and run it on both iOS and Android.
2. How is React Native different from React?
Answer:
FeatureReact (Web)React Native (Mobile)
PlatformBrowseriOS & Android
RenderingHTML & CSSNative Components
NavigationReact RouterReact Navigation
3. What are the core components of React Native?
Answer:
Some core components include:
-
View (like div)
-
Text (like p)
-
Image (for displaying images)
-
TextInput (for input fields)
-
ScrollView (for scrollable content)
-
FlatList (optimized list rendering)
4. How do you create a React Native project?
Answer:
You can use Expo CLI or React Native CLI:
npx create-expo-app MyApp # Using Expo CLI npx react-native init MyApp # Using React Native CLI
5. How do you run a React Native app?
Answer:
-
With Expo CLI:
npm start
-
With React Native CLI:
npx react-native run-android # For Android npx react-native run-ios # For iOS
6. What is JSX?
Answer:
JSX (JavaScript XML) is a syntax extension of JavaScript used in React and React Native to describe the UI.
const App = () => <Text>Hello, React Native!</Text>;
7. How do you style components in React Native?
Answer:
You use the StyleSheet API or inline styles:
import { StyleSheet, Text, View } from 'react-native'; const App = () => ( <View style={styles.container}> <Text style={styles.text}>Hello, React Native!</Text> </View> ); const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center' }, text: { fontSize: 20, color: 'blue' } });
8. What is Flexbox in React Native?
Answer:
React Native uses Flexbox for layout. Some key properties:
-
flexDirection: row or column
-
justifyContent: center, space-between
-
alignItems: center, stretch
Example:
<View style={{ flexDirection: 'row', justifyContent: 'space-between' }}> <Text>Item 1</Text> <Text>Item 2</Text> </View>
9. How do you handle user input in React Native?
Answer:
Use the TextInput component:
const [text, setText] = useState(''); <TextInput value={text} onChangeText={setText} placeholder="Enter text" />;
10. How do you handle button clicks?
Answer:
Use the Button component:
<Button title="Click Me" onPress={() => alert('Button Clicked!')} />
11. How do you navigate between screens in React Native?
Answer:
Use React Navigation:
npm install @react-navigation/native
import { createNativeStackNavigator } from '@react-navigation/native-stack'; const Stack = createNativeStackNavigator(); <Stack.Navigator> <Stack.Screen name="Home" component={HomeScreen} /> <Stack.Screen name="Details" component={DetailsScreen} /> </Stack.Navigator>;
12. How do you use state in React Native?
Answer:
Use the useState hook:
const [count, setCount] = useState(0); <Button title="Increase" onPress={() => setCount(count + 1)} />;
13. What is the difference between useState and useEffect?
Answer:
-
useState manages component state.
-
useEffect runs side effects (e.g., API calls, event listeners).
useEffect(() => { console.log('Component Mounted'); }, []);
14. How do you fetch data from an API in React Native?
Answer:
Use fetch or axios:
useEffect(() => { fetch('https://jsonplaceholder.typicode.com/posts') .then(response => response.json()) .then(data => console.log(data)); }, []);
15. How do you use FlatList in React Native?
Answer:
<FlatList data={[{ id: '1', name: 'Item 1' }, { id: '2', name: 'Item 2' }]} renderItem={({ item }) => <Text>{item.name}</Text>} keyExtractor={item => item.id} />
16. How do you add icons in React Native?
Answer:
Use react-native-vector-icons:
npm install react-native-vector-icons
import Icon from 'react-native-vector-icons/FontAwesome'; <Icon name="home" size={30} color="blue" />;
17. How do you store data locally?
Answer:
Use AsyncStorage:
npm install @react-native-async-storage/async-storage
await AsyncStorage.setItem('username', 'John'); const name = await AsyncStorage.getItem('username');
18. How do you handle gestures in React Native?
Answer:
Use the react-native-gesture-handler library.
npm install react-native-gesture-handler
import { GestureHandlerRootView } from 'react-native-gesture-handler';
19. How do you detect device orientation?
Answer:
Use useWindowDimensions():
const { width, height } = useWindowDimensions(); const isPortrait = height > width;
20. How do you add animations in React Native?
Answer:
Use the Animated API:
const fadeAnim = useRef(new Animated.Value(0)).current; Animated.timing(fadeAnim, { toValue: 1, duration: 1000 }).start();
21. How do you debug a React Native app?
Answer:
Use React Developer Tools, console.log(), and React Native Debugger.
22. How do you handle forms in React Native?
Answer:
Use react-hook-form:
npm install react-hook-form
23. What are React Native lifecycle methods?
Answer:
-
Mounting: componentDidMount
-
Updating: componentDidUpdate
-
Unmounting: componentWillUnmount
24. What is Expo in React Native?
Answer:
Expo is a toolchain for faster React Native development with pre-configured libraries.
25. What are some popular React Native libraries?
Answer:
-
Navigation: react-navigation
-
State Management: Redux, Zustand
-
Animations: react-native-reanimated
-
Storage: AsyncStorage, MMKV