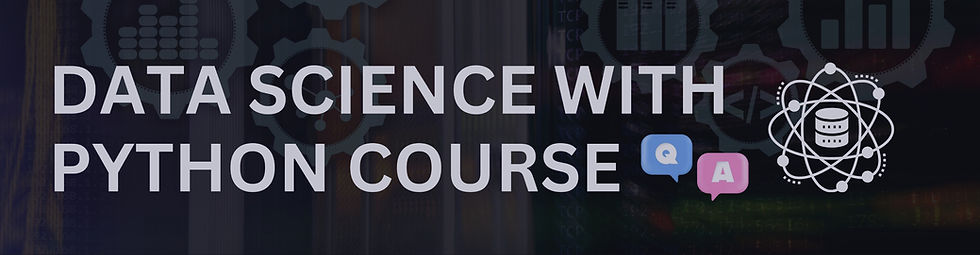
Data Science with Python Interview Questions and Answers
Top 100 Data Science with Python Interview Questions for Freshers
Data Science with Python is one of the most in-demand skills in top tech companies, including IDM TechPark. Mastering both the theoretical concepts and practical applications in Python, along with data analysis tools, machine learning algorithms, and deployment strategies, makes a Python Data Scientist a valuable asset in modern software development. To secure a Data Scientist role at IDM TechPark, candidates must be proficient in technologies like Python, Pandas, NumPy, Scikit-Learn, TensorFlow, Keras, SQL, and cloud services, as well as be ready to tackle both the Data Science with Python Online Assessment and Technical Interview Round.
To help you succeed, we have compiled a list of the Top 100 Data Science with Python Interview Questions along with their answers. Mastering these will give you a strong edge in cracking Data Science interviews at IDM TechPark.
1. What is Data Science?
Answer: Data Science is a field that combines statistical techniques, programming skills, and domain knowledge to extract insights from structured and unstructured data.
2. Why is Python used in Data Science?
Answer: Python is popular in Data Science because of its simplicity, rich libraries (NumPy, Pandas, Matplotlib, etc.), and strong community support.
3. What are some important Python libraries for Data Science?
Answer: Key libraries include:
-
NumPy (numerical computing)
-
Pandas (data manipulation)
-
Matplotlib & Seaborn (data visualization)
-
Scikit-learn (machine learning)
-
TensorFlow & PyTorch (deep learning)
4. What is NumPy and how is it useful?
Answer: NumPy (Numerical Python) provides support for multi-dimensional arrays and mathematical functions essential for data processing.
5. How is Pandas used in Data Science?
Answer: Pandas provides data structures like DataFrames and Series for easy data manipulation and analysis.
6. What is a DataFrame in Pandas?
Answer: A DataFrame is a 2D labeled data structure, similar to a table in SQL or Excel.
7. How do you read a CSV file using Pandas?
Answer:
import pandas as pd df = pd.read_csv('file.csv') print(df.head())
8. What is the difference between Series and DataFrame in Pandas?
Answer:
-
Series is a 1D labeled array.
-
DataFrame is a 2D labeled table with rows and columns.
9. How do you handle missing data in Pandas?
Answer: Use methods like:
df.dropna() # Remove missing values df.fillna(0) # Replace missing values with 0
10. What is Matplotlib used for?
Answer: Matplotlib is a plotting library used to create static, animated, and interactive visualizations in Python.
11. How do you create a simple plot using Matplotlib?
Answer:
import matplotlib.pyplot as plt x = [1, 2, 3, 4] y = [10, 20, 25, 30] plt.plot(x, y) plt.show()
12. What is Seaborn, and how is it different from Matplotlib?
Answer: Seaborn is built on top of Matplotlib and provides better visualizations with more aesthetic styling options.
13. What is Scikit-learn used for?
Answer: Scikit-learn is a machine learning library for Python that provides tools for classification, regression, clustering, and more.
14. How do you split a dataset into training and testing sets using Scikit-learn?
Answer:
from sklearn.model_selection import train_test_split X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
15. What is a confusion matrix?
Answer: A confusion matrix is used to evaluate the performance of a classification model by comparing actual vs. predicted values.
16. What is the difference between Supervised and Unsupervised Learning?
Answer:
-
Supervised Learning: Data has labeled outputs (e.g., regression, classification).
-
Unsupervised Learning: Data has no labeled outputs (e.g., clustering, association).
17. What is a Linear Regression model?
Answer: Linear regression is a supervised learning algorithm used for predicting a continuous target variable based on input features.
18. How do you implement Linear Regression in Python?
Answer:
from sklearn.linear_model import LinearRegression model = LinearRegression() model.fit(X_train, y_train)
19. What is Logistic Regression?
Answer: Logistic regression is a classification algorithm used for binary or multi-class classification problems.
20. What is Overfitting and Underfitting?
Answer:
-
Overfitting: Model learns too much from training data and performs poorly on new data.
-
Underfitting: Model is too simple and fails to capture patterns in data.
21. What is Cross-validation?
Answer: Cross-validation is a technique used to improve model performance by splitting data into multiple training/testing sets.
22. How do you normalize data in Python?
Answer:
from sklearn.preprocessing import MinMaxScaler scaler = MinMaxScaler() X_scaled = scaler.fit_transform(X)
23. What is Feature Engineering?
Answer: Feature engineering is the process of selecting, transforming, or creating new features to improve model performance.
24. How do you remove duplicate values in Pandas?
Answer:
df.drop_duplicates(inplace=True)
25. What is the difference between .loc[] and .iloc[] in Pandas?
Answer:
-
.loc[]: Used for label-based indexing.
-
.iloc[]: Used for position-based indexing.